C++ program to find odd numbers between 1 to 100
// In this C++ program the for loop is generating a number from 1 to 100
// and increment by 1. In loop the program will check each number whether
// it is odd or not by taking a%2!=0. If result of a mod 2 is not equals to 0
// means number is odd and it will get printed by cout statement
#include<iostream.h>
#include<conio.h>
main()
{
clrscr();
int a;
for (a=1;a<=100;a++)
{
if (a%2!=0)
{
cout << a <<endl;
}
}
getch();
}
Feb 27, 2007
C++ program to find odd numbers between 1 to 100
C++ program to find even numbers between 1 to 100
// and increment by 1. In loop the program will check each number whether
// it is even or not by taking a%2==0. If result of a mod 2 is equals to 0
// means number is even and it will get printed by cout statement
#include<iostream.h>
#include<conio.h>
main()
{
clrscr();
int a;
for (a=1;a<=100;a++)
{
if (a%2!=0)
{
cout << a <<endl;
}
}
getch();
}
Feb 26, 2007
C++ program to generate a melodious sound from PC speaker
#include<iostream.h>
#include<conio.h>
#include<dos.h>
main()
{
clrscr();
for(int i=100;i<=1000;i=i+100)
{
sound(i);
delay(500); // time delay in milliseconds
nosound(); // it will stop the sound
}
getch();
}
C++ program to generate a beep sound from the PC speaker
#include<iostream.h>
#include<conio.h>
#include<dos.h>
main()
{
clrscr();
sound(500); // 500 is frequency
delay(1000); // time delay in milliseconds
nosound(); // it will stop the sound
getch();
}
Feb 24, 2007
Creating picture package in Photoshop: (Tutorial)
Scanning a photograph:
Step 1: Scan the photograph for which you want to make a picture package by your scanner and save the scanned image file.
Step 2: Open the scanned image file in Photoshop.
Resizing the image:
Step 3: Now change the image size by clicking “Image menu > Image size”. Image size dialogue box will be appeared on the screen. Set the Document size type in width as 2 and height as 3 inches and set the resolution as 200. Before doing this also uncheck the Constrain Proportion checkbox and then press “Ok” button to resize the image. For more details on how to resize the image click here and read. After resizing the image your image should look like this.
Making a border around the photo:
Step 4: Now Click “Select menu > All” to select the entire image. Now click “Edit > Stroke”, Stroke dialogue box will be appeared on the screen. Step 5: Now type in stroke width 5 px and choose Color of stroke as white and choose Stroke Location is “Inside” and Blending mode is normal, Opacity is 100% and then press “Ok” button. Now the image will be looked like the following picture.
Defining a pattern of image:
Step 6: Now click “Select > All” to select the entire image again and then click “Edit > Define Pattern”. “Define Pattern” dialogue box will be appeared on the screen. It asks for name of the pattern which you want to create. Type in a desired name there and press “Ok” button to close the window. Now we have successfully created a pattern.Creating Package of defined pattern:
Step 7: Now click “File > New” to create a new file. New file dialogue box will be appeared on the screen set width as 8 inches and height as 12 inches and resolution of the image as 200 pixels/inch and then press “Ok” button. A blank 8x12 inches page will be appeared on the screen.Here you may see that we have created a blank page of size 8 width and 12 height and our image size is 2x3 means 2 inches width and 3 inches height so that photo array will be 4x4 means total 16 passport photos. By this way you can calculate the measurement by your requirement and alter the above sizes.
Step 8: Click “Edit > Fill” then Fill dialogue box will be appeared on the screen. Choose “Pattern” from the Use drop down list box and Choose your define pattern from Custom pattern drop down list box. You may find newly created pattern at the end of the custom pattern list. Leave the opacity to 100% and Blending mode as normal and then press “Ok” button to fill the created pattern in blank page and you are done.Hope that you will like this method. If you face any difficulty you can feel free to mail me.
Read other article to create picture package in Photoshop.
Rotating Canvas in Photoshop:
Rotating an image 180 degree:
Step 1: To rotate an image click “Image menu > Rotate Canvas > 180. Image would be upside down as shown in the following picture. This should be helpful to you if you scanned an image upside down. So by rotating 180 degree problem will be solved.
After Rotating an image 180 Degree
Rotating an image 90 degree CW:
To do so click “Image menu> Rotate Canvas > 90 CW”.
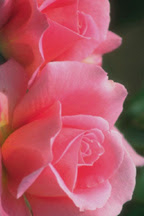
After Rotating an image 90 Degree Clockwise
Rotating an image 90 degree CCW:
To do so click “Image menu> Rotate Canvas > 90 CCW”
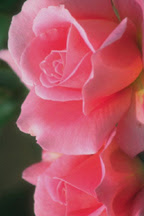
After Rotating an image 90 Degree Counter Clockwise
Rotating an image arbitrary:
To do so click “Image menu > Rotate Canvas > Arbitrary”. A dialogue box will be appeared on the screen as shown below.
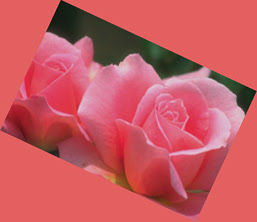
Flipping Image Horizontally:
To do so click “Image menu> Rotate Canvas> Flip Horizontal”. Flipping Image Vertically:
To do so click “Image menu> Rotate Canvas>Flip Vertical”.
Feb 23, 2007
Creating Backgrounds in Photoshop: (Tutorial)
You can create special background for your photograph using Fill option in Photoshop.
Step 1: Start Photoshop.
Step 2: Click “File > New’. New Dialogue box will be appeared on the screen.
* Name of the Image file
* Size of the image in width and height
* Resolution of the image
* Mode of colors
* Content in the image file
Name Of the Image File:
In name textbox type in an appropriate name of the image file you want.
Size of the image in width and height:
You can also specify size of the image in width and height by typing in their textboxes. Size of the image could be in inches, cm, pixels etc.
Resolution of the image:
Here you can specify resolution of the image whether it is 300, 400 or 600 choose the appropriate one.
Mode of colors:
Choose RGB color from the drop down list box.
Content in the image file:
In Content drop down, Choose “White” if you want to fill background with white. Choose “Background Color” if you want to fill the new file with Background color. This background color can be set from the toolbox as shown below.
When you click “set background color” button as marked in the above picture to change the background color a “Color Picker” dialogue box will be appeared on the screen. Choose the appropriate color for your background and then press “Ok” button to close the Color Picker dialogue box.
Step 3: then press “OK” button.
"White" Background Fill
"Backgroud Color" Background Fill
"Transparent" Background Fill
Step 4: Click “Edit > Fill”. Fill Dialogue box will be appeared on the screen.There are many option that you can fill in the background of your image.
You can fill
Foreground color
Background color
Pattern
History
Black
50% Gray
White
Foreground and Background colors are which you set from the toolbox.
Step 5: Now choose “Pattern” from the “use” drop down list box. Custom pattern drop down list box will get activate. Now choose any one pattern of your choice from the list. And then choose “Mode” as normal. Now you can specify opacity in percentage. What actually opacity is. Opacity means if you specify 100%, then background would be 100% opaque and 0% opacity means the background would be completely transparent.
Step 6: Leave opacity at 100% and press “Ok” button. Now try with different opacity and do the experiment with fill.
You can change custom patterns by doing the following steps.
Click “Edit > Fill > Custom Pattern”. Now click the button as shown in the following picture.A popup menu will be appeared on the screen choose “pattern2.pat” or “patterns.pat” from the popup menu. A confirmation window will be appeared on the screen press “Ok” to load the pattern in the custom pattern.
Now you can use newly loaded custom patterns for your background. We can also create user defined patterns in Photoshop by defining new patterns. I will write on this in my forthcoming post.
Technorati Tags : Photoshop, Background , Create , Fill
Feb 22, 2007
Sharpen Filter in Photoshop
Step 1: Open photo in Photoshop which is out of focus.
Origional Image
Step 2: Then click “Filter > Sharpen > Sharpen. The image will be sharp. If you need more sharpen then click “Filter > Sharpen > Sharpen More”. After applying sharpen effect image will be looked like this.
After Applying Sharpen More Filter
Step 3: Click “Filter > Sharpen > Sharpen Edges” then only edges of the image will be get shaped as shown in the following picture.
After Applying Sharpen Edges Filter
Changing canvas size in Photoshop
Step 1: open the scanned image or snap you have taken by your digital camera.
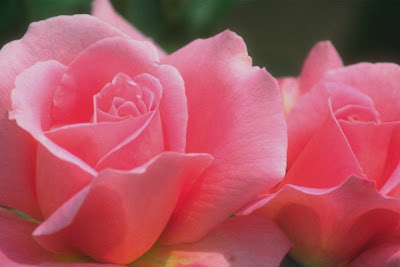
Step 2: Click “Image > Canvas Size”. “Canvas size” dialogue box will be appeared on the screen.
Step 3: Type 13 as a width and 10 as a height. Now the will be looked like this.
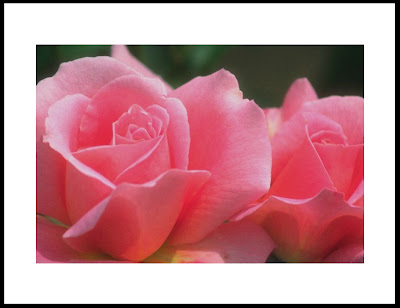
Anchor shows that where you want to put the image after changing the size of the canvas. You can also set the position of the photo by clicking desired anchor point. But by default it is set to the center.
Step 4: Change the anchor point to upper left corner so the image will be place on the upper left corner.
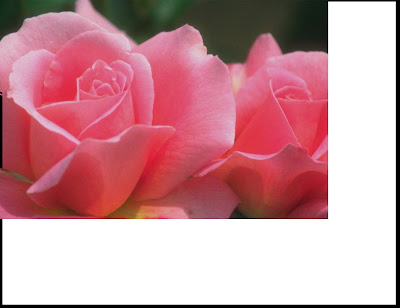
Technorati Tags : Photoshop, Size ,Canvas ,How to
Feb 21, 2007
Color photograph into Grayscale in Photoshop :
Step 2: then click “Image > Mode > Grayscale”. A confirmation window will be appeared on the screen. It will ask you that all color information in the photo will be discarded.
Step 3: Press “Ok” button and you are done. Your color photograph will be converted in a Grayscale image.
Adjusting Brightness and Contrast in Photoshop: (Tutorial)
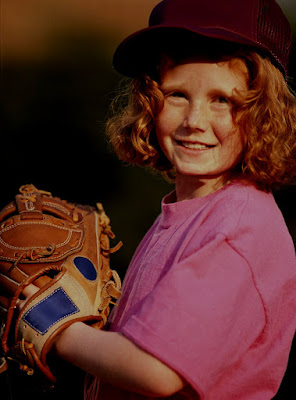
Before Adjusting Brightness/Contrast
Click image > Adjust > Brightness/Contrast. “Brightness/Contrast” window will be appeared on the screen. Now adjust the brightness/contrast slider of the window. Also make sure that “Preview” Checkbox is checked. See the result when you are adjusting brightness/contrast then press “Ok” button when you are done.
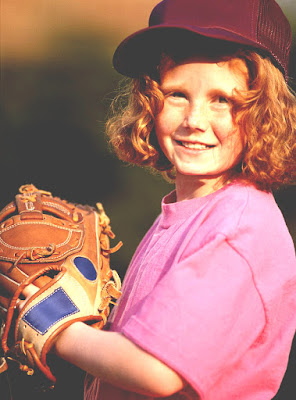
After Adjusting Brightness/Contrast
Also there is an option to set the contrast of an image automatically. For that click “Image>Adjust>Auto Contrast”. Use this feature if you don’t have enough time to set the Brightness/Contrast of a picture.
Technorati Tags: Photoshop ,Photo , Contrast, Brightness, How to
How To Resize Picture in Photoshop (Enlarge or Reduce Images):
Step 1: Open the photo file, which is to be reduced or enlarged in Photoshop.
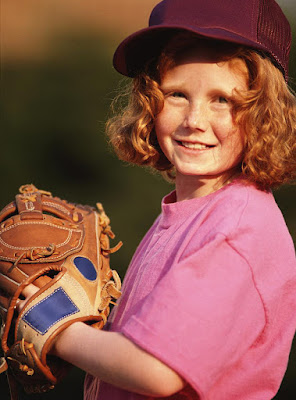
Step 3: “Image Size” dialogue box will be appeared on the screen.
“Image Size” dialogue box shows size of image. In the case of above picture Its pixel dimension shows width of the image is “888” pixels and height of the image is “1200” pixels. This image size can be changed.
Step 4: Change its width to “400” pixels by typing in its width text box. Only width of image will be changed as shown in the following photo.
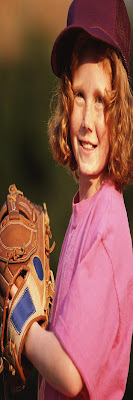
If we want to change size of the photo in proportion, check the “Constrain Proportion” Check box. Then change the size o the photo, now photo will be resized in proportion.
In “Image size” dialogue box, you can also set resolution of the image.
Technorati Tags : Photoshop ,Resize , Photo, How to